CSS selectors
- At this point, we only know the basic CSS selectors
- Simple selectors, based on (element) name, id and class
p {
text-align: center;
color: red;
}
h1, h2 {
text-transform: uppercase;
}
#container {
max-width: 600px;
margin: auto;
}
.underline {
text-decoration: underline;
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
- Descendant selector (
space
), e.g. all paragraphs within an article
element
article p {
line-height: 1.5;
}
1
2
3
- There are a lot more powerful selectors available for us
Combinator selectors
- Select elements based on a specific relationship between them (using
space
, >
, +
, ~
)

selector | description |
div p | descendant selector: selects all p elements inside div elements |
div > p | child selector: selects all p elements that are the children of a div element |
div + p | adjacent sibling selector: selects every p element that is placed immediately after a div element (same level) |
div ~ p | general sibling selector: selects every p element that is preceded by a div element (same level) |
Pseudo-class selectors
- Select elements based on a certain state (
:state
)
selector | description |
a:hover | selects the a element that is hovered over |
input:focus | selects the input element that has focus |
input:valid | selects every input element that has a valid value |
input:invalid | selects every input element that has an invalid value |
p:first-child | selects every p element that is the first child of its parent |
p:first-of-type | selects every p element that is the first p element of its parent |
p:nth-child(2) | selects every p element that is the second child of its parent |
p:nth-of-type(2) | selects every p element that is the second p element of its parent |
... | ... |
Pseudo-element selectors
- Select and style a part of an element
selector | description |
p::first-letter | selects the first letter of each p element |
p::first-line | selects the first line of each p element |
p::selection | selects the portion of the p element that is selected by a user |
p::after | insert something after the content of each p element |
p::before | insert something before the content of each p element |
... | ... |
Attribute selectors
- Select elements based on an attribute or attribute value
selector | description |
label[for] | selects all label elements with the for attribute |
input[type="submit"] | selects all input elements where the type attribute equals submit |
a[href^="https"] | selects every a element whose href attribute value begins with https |
a[href$=".pdf"] | selects every a element whose href attribute value ends with .pdf |
[id*="test"] | selects every element whose id attribute value contains the substring test |
... | ... |
REMARK: JavaScript/jQuery
- We discussed these selectors in the context of selecting elements and styling them using CSS
- These selectors can also be used to select elements in JavaScript to make our website more dynamic
- The better your knowledge about selectors, the better you can select the elements you want and the less code you have to write
- More about this on the ES6 and jQuery pages!
Learn on the go
- You can play around with selectors in our dynamic example (opens new window)
- Write a selector in the 'selector' input field and see the result immediately!
- JavaScript is used to select the elements and give them the CSS class
outline
, which results in the yellow background and red border
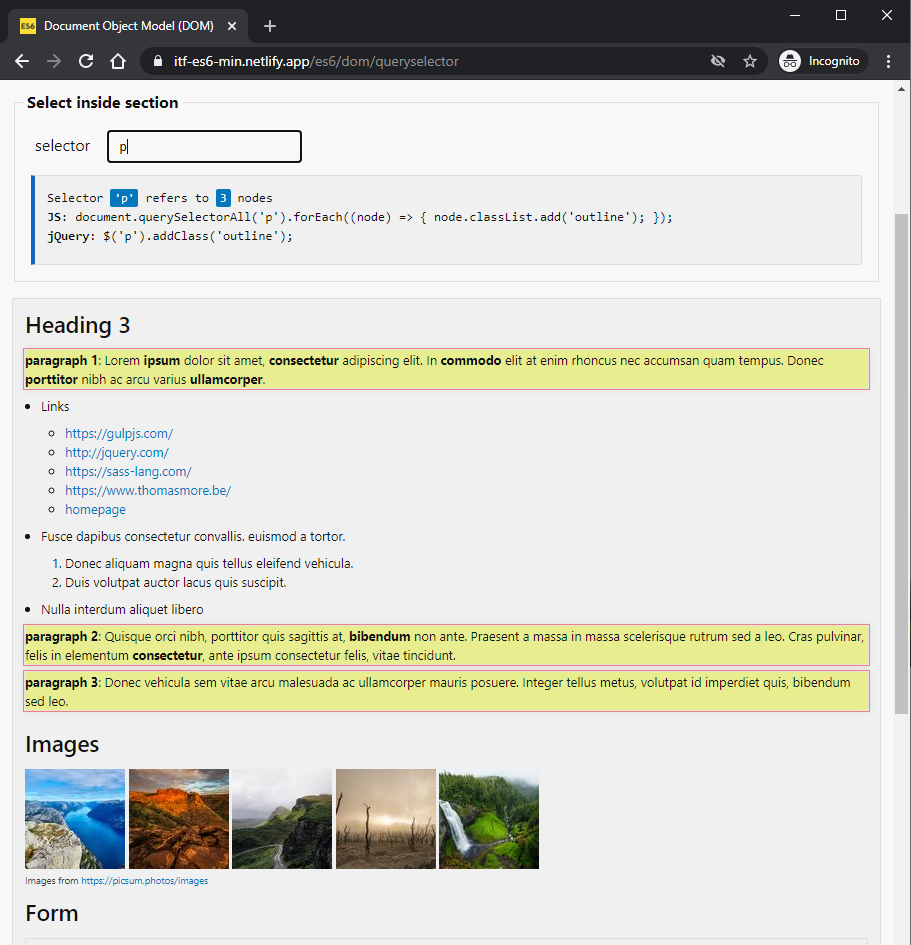